When an error occurs with an app Created with Create React App the error screen is super helpful.
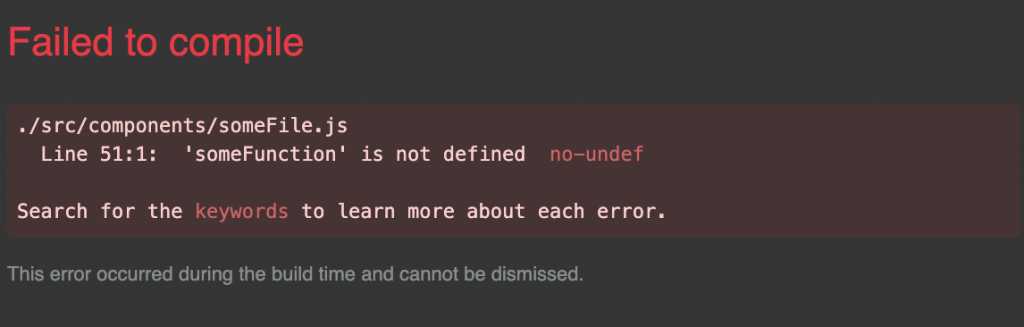
You can click on this error in the browser to jump directly to the line of code inside your editor! But how do you setup VSCode with REACT_EDITOR?
Console error:
Could not open SomeFile.js in the editor.
To set up the editor integration, add something like REACT_EDITOR=atom to the .env.local file in your project folder and restart the development server. Learn more: https://goo.gl/MMTaZt
Setup your editor to be open from terminal
Google “How to open [your editor] from terminal” to find instructions for your editor.
For VSCode, open the command pallet ( Command Key + P ) and type > shell
to install “code” into your PATH environment variables.

You should now be able to open terminal and type code
to open VSCode. Pretty nifty!
Setup your React Project to know which editor to open
Create a new file (or edit the existing) .env.local
file.
setup VSCode with REACT_EDITOR this will be REACT_EDITOR=code
and for other editors set it to the command you found to open your editor from the terminal.
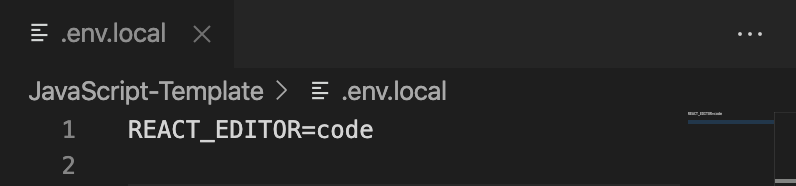
Credit to this StackOverflow Answer.
Related post: Favorite Sublime 3 Packages
(cover photo credit: Jonathan Stassen / JStassen Photography)